티스토리 뷰
Stack Overflow에 자주 검색, 등록되는 문제들과 제가 개발 중 찾아 본 문제들 중에서 나중에도 찾아 볼 것 같은 문제들을 정리하고 있습니다.
Stack Overflow에서 가장 먼저 확인하게 되는 가장 높은 점수를 받은 Solution과 현 시점에 도움이 될 수 있는 가장 최근에 업데이트(최소 점수 확보)된 Solution을 각각 정리하였습니다.
아래 word cloud를 통해 이번 포스팅의 주요 키워드를 미리 확인하세요.
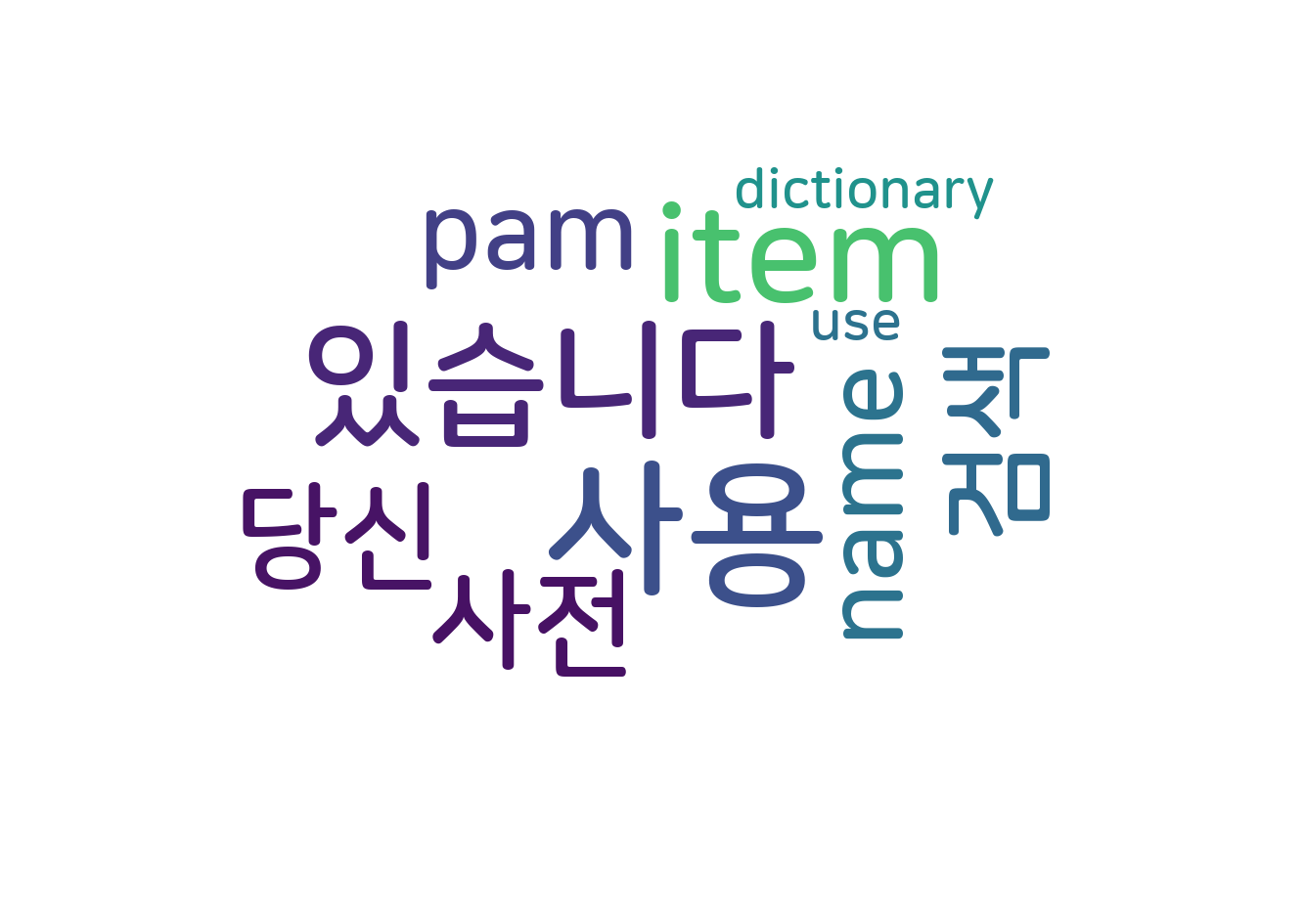
Python list of dictionaries search
Python 딕셔너리 검색 리스트
문제 내용
Assume I have this:
제가 이걸 가지고 있다고 가정해보세요:
[
{"name": "Tom", "age": 10},
{"name": "Mark", "age": 5},
{"name": "Pam", "age": 7}
]
and by searching "Pam" as name, I want to retrieve the related dictionary: {name: "Pam", age: 7}
그리고 "팸"을 이름으로 검색하여 관련 딕셔너리를 검색합니다. {name: "팸", 나이: 7}
How to achieve this ?
이를 달성하는 방법은 무엇입니까?
높은 점수를 받은 Solution
You can use a generator expression:
제너레이터 식을 사용할 수 있습니다.
>>> dicts = [
... { "name": "Tom", "age": 10 },
... { "name": "Mark", "age": 5 },
... { "name": "Pam", "age": 7 },
... { "name": "Dick", "age": 12 }
... ]
>>> next(item for item in dicts if item["name"] == "Pam")
{'age': 7, 'name': 'Pam'}
If you need to handle the item not being there, then you can do what user Matt suggested in his comment and provide a default using a slightly different API:
만약 당신이 그곳에 없는 아이템을 처리해야 한다면, 당신은 사용자 Matt가 그의 코멘트에서 제안한 것을 하고 약간 다른 API를 사용하여 기본값을 제공할 수 있습니다.
next((item for item in dicts if item["name"] == "Pam"), None)
And to find the index of the item, rather than the item itself, you can enumerate() the list:
또한 아이템 자체가 아닌 아이템의 인덱스를 찾으려면 리스트를 enumerate() 하여 구할 수 있습니다.
next((i for i, item in enumerate(dicts) if item["name"] == "Pam"), None)
가장 최근 달린 Solution
Put the accepted answer in a function to easy re-use
쉽게 재사용할 수 있도록 승인된 답변을 함수에 넣으세요.
def get_item(collection, key, target):
return next((item for item in collection if item[key] == target), None)
Or also as a lambda
또는 람다로도 사용할 수 있습니다.
get_item_lambda = lambda collection, key, target : next((item for item in collection if item[key] == target), None)
Result
결과
key = "name"
target = "Pam"
print(get_item(target_list, key, target))
print(get_item_lambda(target_list, key, target))
#{'name': 'Pam', 'age': 7}
#{'name': 'Pam', 'age': 7}
In case the key may not be in the target dictionary use dict.get and avoid KeyError
키가 대상 딕셔너리에 없을 경우 dict.get을 사용하여 KeyError를 방지하세요.
def get_item(collection, key, target):
return next((item for item in collection if item.get(key, None) == target), None)
get_item_lambda = lambda collection, key, target : next((item for item in collection if item.get(key, None) == target), None)
출처 : https://stackoverflow.com/questions/8653516/python-list-of-dictionaries-search
'개발 > 파이썬' 카테고리의 다른 글
데이터프레임 행 순서 무작위로 섞기 (0) | 2022.12.17 |
---|---|
특정 키만 포함하도록 딕셔너리를 필터링하기 (0) | 2022.12.17 |
파이썬에서 파일에 대한 flush 빈도 (0) | 2022.12.17 |
부분 문자열 기준으로 데이터프레임 필터링 하기 (0) | 2022.12.16 |
파일의 첫 번째 줄 읽어오기 (0) | 2022.12.16 |